SharePoint PowerShell: Upload multiple documents to sharepoint document library
Hi,
I was creating a script to replicate document library structure similar to Prod just in order to test the ShareGate Migration. Let me take a brief description about my scenario:
Description:
There is a document library present on Prod, which contains bulk document (~3000). Now I need to update metadata for all those documents using Excel sheet and ShareGate. I don't have access to the Production library and not even replicate the Library to my Dev environment as it contains some very confidential documents.
Solution:
I have decided to create a script which will take one template document from file system and read name of document from one of columns of excel. The script will take some time(~hours in case of thousand documents) to upload the template with the new file name. The inputs to the script will be
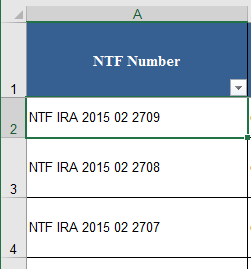
Code:
Output:

Hope that code help you finding solution.
Happy SharePointing :)
Mohit Vashishtha
I was creating a script to replicate document library structure similar to Prod just in order to test the ShareGate Migration. Let me take a brief description about my scenario:
Description:
There is a document library present on Prod, which contains bulk document (~3000). Now I need to update metadata for all those documents using Excel sheet and ShareGate. I don't have access to the Production library and not even replicate the Library to my Dev environment as it contains some very confidential documents.
Solution:
I have decided to create a script which will take one template document from file system and read name of document from one of columns of excel. The script will take some time(~hours in case of thousand documents) to upload the template with the new file name. The inputs to the script will be
- Path of the excel which contains name of documents in one column
- Path of the file where the template is placed on file system
- Url of the sharepoint web site
- Name of the document library
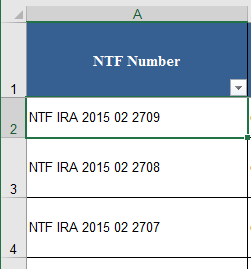
Code:
# Add SharePoint Snapin to PowerShell
if((Get-PSSnapin | Where {$_.Name -eq "Microsoft.SharePoint.PowerShell"}) -eq $null)
{
Add-PSSnapin Microsoft.SharePoint.PowerShell
}
$exlFile = "D:\Work\Book2 - Copy.xlsx"
$fileExt = ".pdf"
#$filePath = "D:\Work\IAEA.docx"
$filePath = "D:\Work\pdf.pdf"
$webUrl = "http://server/sites/site"
$docLibName = "Library Name"
try {
$xl = New-Object -COM "Excel.Application"
#$xl.Visible = $true
$wb = $xl.Workbooks.Open($exlFile)
#$ws = $wb.Sheets.Item('Log')
$ws = $wb.Sheets.Item('Sheet1')
$lines = $ws.UsedRange.Rows.Count
$spWeb = Get-SPWeb -Identity $webUrl
$spWeb.AllowUnsafeUpdates = $true;
$lib = $spWeb.Lists[$docLibName]
$folder = $lib.RootFolder
#$sourceFileName = $filePath.Substring($filePath.LastIndexOf("\")+1)
$file= Get-ChildItem $filePath
$docLibraryUrl = $lib.RootFolder.ServerRelativeUrl
$uploadfolder = $spWeb.getfolder($docLibraryUrl + $folderPathWithinDocLibrary)
$fileStream = ([System.IO.FileInfo] (Get-Item $file.FullName)).OpenRead()
for ($line = 2; $line -le $lines; $line ++) {
$fileName = $ws.Columns.Item(1).Rows.Item($line).Text
if($fileName -ne "")
{
$fileName
#Check if File exist
$item = $uploadfolder.Files | where{$_.Name -eq $fileName}
if(!$item)
{
#Add file
write-host -NoNewLine -f yellow "Addin file " $fileName " to " $folder.ServerRelativeUrl "..."
$newFilePath = $docLibraryUrl + $folderPathWithinDocLibrary + "/" + $fileName
write-host "Creating document: $newFilePath ..."
$spFile = $uploadfolder.Files.Add($newFilePath, [System.IO.Stream]$fileStream, $true)
write-host -f Green "...Success!"
}
}
}
#Close file stream
$fileStream.Close()
$spWeb.AllowUnsafeUpdates = $false;
$spWeb.Dispose();
$xl.Quit()
}
finally {
$wb.Close()
$xl.Quit()
[System.Runtime.Interopservices.Marshal]::ReleaseComObject($xl)
}
Output:

Hope that code help you finding solution.
Happy SharePointing :)
Mohit Vashishtha
Comments
Post a Comment